Ios Simulator Macos
The first step is downloading Xcode and installing the iOS simulator on your Mac. Here’s how to install the iOS simulator on your Mac: Download and install Xcode from the Mac App Store. Right click on the Xcode icon in the Applications folder and select Show Package Contents, as shown below. Open the iPhone Simulator application. Yes, it’s completely possible. Assuming your VM is set up, open up the Mac App Store. Install Xcode from the Mac App Store, it’s free, but it’s a big download, so make sure you have something to do while it’s downloading. After I updated macOS on macbook with M1, my project crashes when running on any iOS simulator (runs correctly on physical devices). I am using firebase, GoogleSignIn and FacebookLogin as my pod packages. I noticed that other projects without pods run correctly on simulators. For mac, it comes with an app simulator for iOS, watch/iMessage/tvOS.This Xcode is free for download, and one of the best apps for mac users and developers. Electric Mobile Studio. This emulator gives you a web kit and chrome debugging tool so you can test your web apps. You get a full-fledged emulation for IOS devices and other applications. Search for iOS simulator using the Search app. As for me, this is the easiest way. I use Alfred, but you can use the standard Mac application Spotlight Search.
-->In this tutorial, you build an iOS or macOS app that integrates with the Microsoft identity platform to sign users and get an access token to call the Microsoft Graph API.
When you've completed the guide, your application will accept sign-ins of personal Microsoft accounts (including outlook.com, live.com, and others) and work or school accounts from any company or organization that uses Azure Active Directory. This tutorial is applicable to both iOS and macOS apps. Some steps are different between the two platforms.
In this tutorial:
- Create an iOS or macOS app project in Xcode
- Register the app in the Azure portal
- Add code to support user sign-in and sign-out
- Add code to call the Microsoft Graph API
- Test the app
Prerequisites
How tutorial app works
The app in this tutorial can sign in users and get data from Microsoft Graph on their behalf. This data will be accessed via a protected API (Microsoft Graph API in this case) that requires authorization and is protected by the Microsoft identity platform.
More specifically:
- Your app will sign in the user either through a browser or the Microsoft Authenticator.
- The end user will accept the permissions your application has requested.
- Your app will be issued an access token for the Microsoft Graph API.
- The access token will be included in the HTTP request to the web API.
- Process the Microsoft Graph response.
This sample uses the Microsoft Authentication Library (MSAL) to implement Authentication. MSAL will automatically renew tokens, deliver single sign-on (SSO) between other apps on the device, and manage the account(s).
If you'd like to download a completed version of the app you build in this tutorial, you can find both versions on GitHub:
- iOS code sample (GitHub)
- macOS code sample (GitHub)
Create a new project
- Open Xcode and select Create a new Xcode project.
- For iOS apps, select iOS > Single view App and select Next.
- For macOS apps, select macOS > Cocoa App and select Next.
- Provide a product name.
- Set the Language to Swift and select Next.
- Select a folder to create your app and select Create.
Register your application
- Sign in to the Azure portal.
- If you have access to multiple tenants, use the Directory + subscription filter in the top menu to select the tenant in which you want to register an application.
- Search for and select Azure Active Directory.
- Under Manage, select App registrations > New registration.
- Enter a Name for your application. Users of your app might see this name, and you can change it later.
- Select Accounts in any organizational directory (Any Azure AD directory - Multitenant) and personal Microsoft accounts (e.g. Skype, Xbox) under Supported account types.
- Select Register.
- Under Manage, select Authentication > Add a platform > iOS/macOS.
- Enter your project's Bundle ID. If you downloaded the code, this is
com.microsoft.identitysample.MSALiOS
. If you're creating your own project, select your project in Xcode and open the General tab. The bundle identifier appears in the Identity section. - Select Configure and save the MSAL Configuration that appears in the MSAL configuration page so you can enter it when you configure your app later.
- Select Done.
Add MSAL
Mac Os Simulator Apk
Choose one of the following ways to install the MSAL library in your app:
CocoaPods
If you're using CocoaPods, install
MSAL
by first creating an empty file calledpodfile
in the same folder as your project's.xcodeproj
file. Add the following topodfile
:Replace
<your-target-here>
with the name of your project.In a terminal window, navigate to the folder that contains the
podfile
you created and runpod install
to install the MSAL library.Close Xcode and open
<your project name>.xcworkspace
to reload the project in Xcode.
Carthage
If you're using Carthage, install MSAL
by adding it to your Cartfile
:
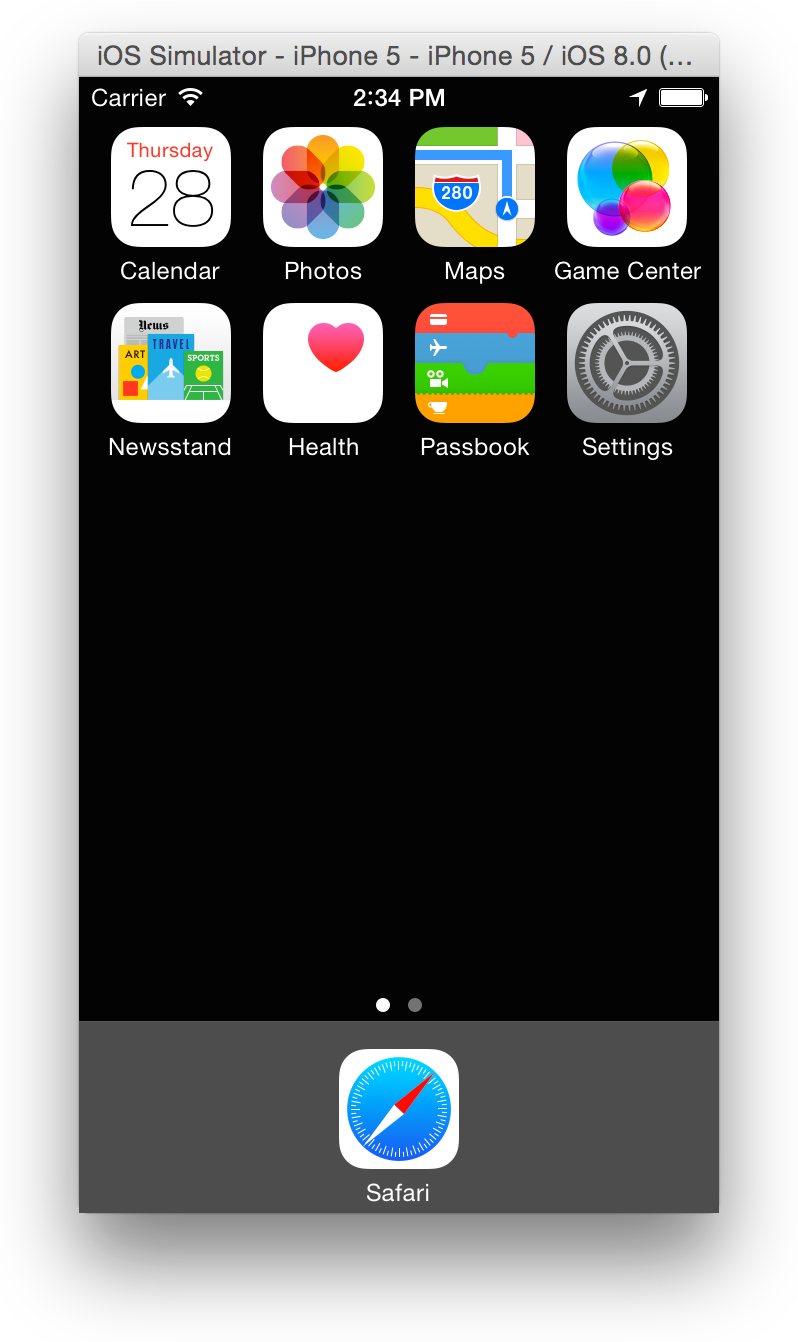
From a terminal window, in the same directory as the updated Cartfile
, run the following command to have Carthage update the dependencies in your project.
iOS:
macOS:
Manually
You can also use Git Submodule, or check out the latest release to use as a framework in your application.
Add your app registration
Next, we'll add your app registration to your code.
First, add the following import statement to the top of the ViewController.swift
, as well as AppDelegate.swift
or SceneDelegate.swift
files:
Then Add the following code to ViewController.swift
prior to viewDidLoad()
:
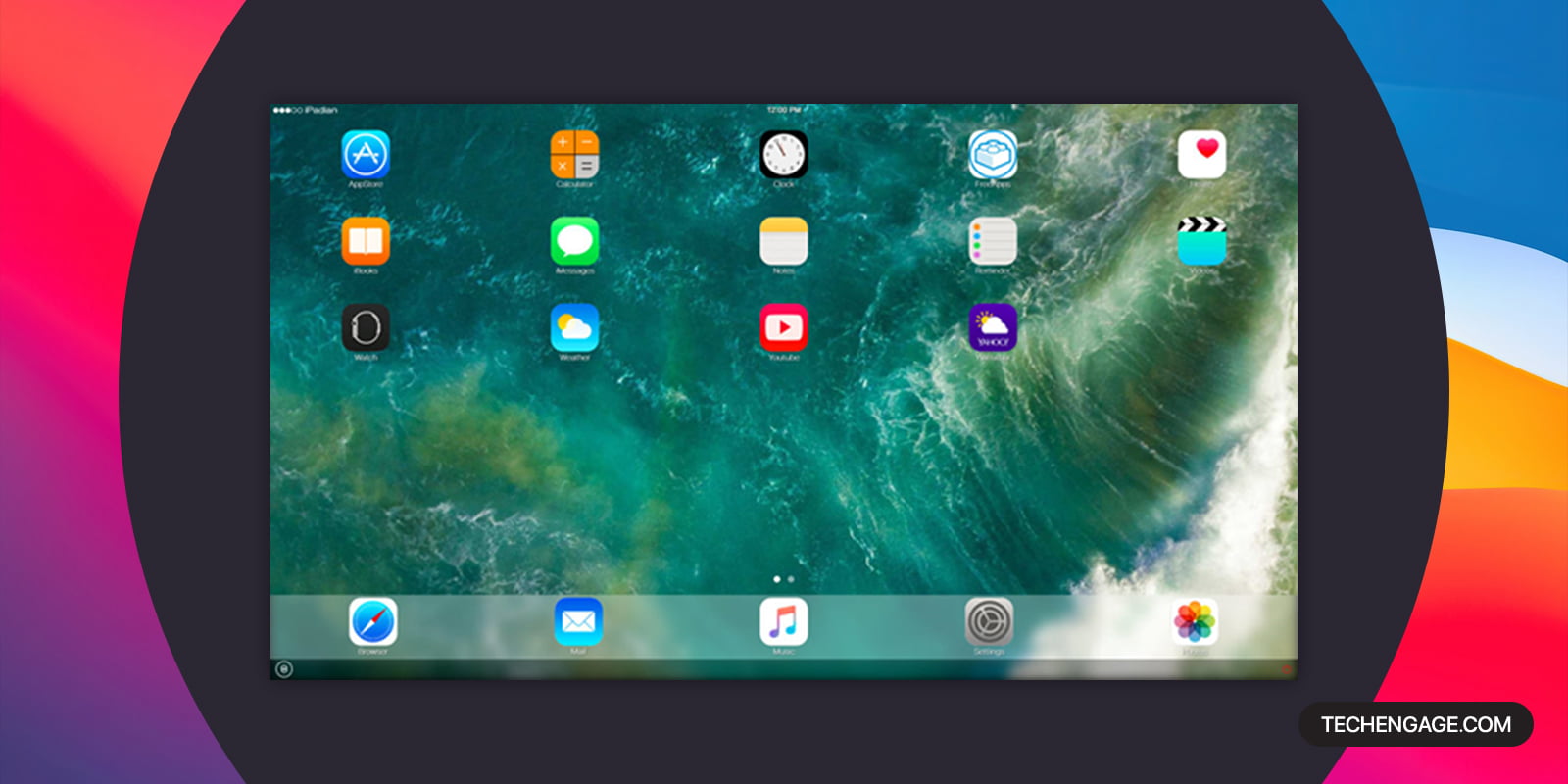
The only value you modify above is the value assigned to kClientID
to be your Application ID. This value is part of the MSAL Configuration data that you saved during the step at the beginning of this tutorial to register the application in the Azure portal.
Configure Xcode project settings
Add a new keychain group to your project Signing & Capabilities. The keychain group should be com.microsoft.adalcache
on iOS and com.microsoft.identity.universalstorage
on macOS.
For iOS only, configure URL schemes
In this step, you will register CFBundleURLSchemes
so that the user can be redirected back to the app after sign in. By the way, LSApplicationQueriesSchemes
also allows your app to make use of Microsoft Authenticator.
In Xcode, open Info.plist
as a source code file, and add the following inside of the <dict>
section. Replace [BUNDLE_ID]
with the value you used in the Azure portal. If you downloaded the code, the bundle identifier is com.microsoft.identitysample.MSALiOS
. If you're creating your own project, select your project in Xcode and open the General tab. The bundle identifier appears in the Identity section.
For macOS only, configure App Sandbox
- Go to your Xcode Project Settings > Capabilities tab > App Sandbox
- Select Outgoing Connections (Client) checkbox.
Create your app's UI
Now create a UI that includes a button to call the Microsoft Graph API, another to sign out, and a text view to see some output by adding the following code to the ViewController
class:
iOS UI
macOS UI
Next, also inside the ViewController
class, replace the viewDidLoad()
method with:
Use MSAL
Initialize MSAL
Add the following initMSAL
method to the ViewController
class:
Add the following after initMSAL
method to the ViewController
class.
iOS code:
macOS code:
For iOS only, handle the sign-in callback
Open the AppDelegate.swift
file. To handle the callback after sign-in, add MSALPublicClientApplication.handleMSALResponse
to the appDelegate
class like this:
If you are using Xcode 11, you should place MSAL callback into the SceneDelegate.swift
instead.If you support both UISceneDelegate and UIApplicationDelegate for compatibility with older iOS, MSAL callback would need to be placed into both files.
Acquire Tokens
Now, we can implement the application's UI processing logic and get tokens interactively through MSAL.
MSAL exposes two primary methods for getting tokens: acquireTokenSilently()
and acquireTokenInteractively()
:
acquireTokenSilently()
attempts to sign in a user and get tokens without any user interaction as long as an account is present.acquireTokenSilently()
requires providing a validMSALAccount
which can be retrieved by using one of MSAL account enumeration APIs. This sample usesapplicationContext.getCurrentAccount(with: msalParameters, completionBlock: {})
to retrieve current account.acquireTokenInteractively()
always shows UI when attempting to sign in the user. It may use session cookies in the browser or an account in the Microsoft authenticator to provide an interactive-SSO experience.
Add the following code to the ViewController
class:
Get a token interactively
The following code snippet gets a token for the first time by creating an MSALInteractiveTokenParameters
object and calling acquireToken
. Next you will add code that:
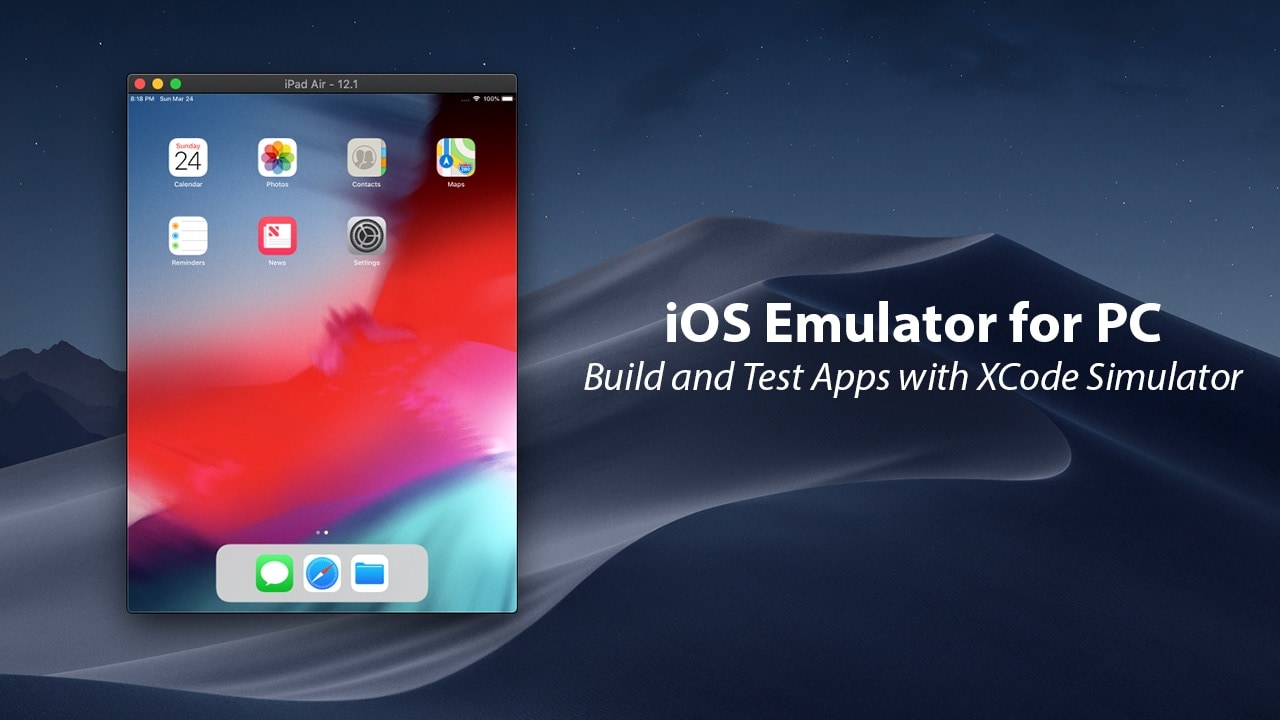
- Creates
MSALInteractiveTokenParameters
with scopes. - Calls
acquireToken()
with the created parameters. - Handles errors. For more detail, refer to the MSAL for iOS and macOS error handling guide.
- Handles the successful case.
Add the following code to the ViewController
class.
The promptType
property of MSALInteractiveTokenParameters
configures the authentication and consent prompt behavior. The following values are supported:
.promptIfNecessary
(default) - The user is prompted only if necessary. The SSO experience is determined by the presence of cookies in the webview, and the account type. If multiple users are signed in, account selection experience is presented. This is the default behavior..selectAccount
- If no user is specified, the authentication webview presents a list of currently signed-in accounts for the user to select from..login
- Requires the user to authenticate in the webview. Only one account may be signed-in at a time if you specify this value..consent
- Requires the user to consent to the current set of scopes for the request.
Get a token silently
To acquire an updated token silently, add the following code to the ViewController
class. It creates an MSALSilentTokenParameters
object and calls acquireTokenSilent()
:
Call the Microsoft Graph API
Once you have a token, your app can use it in the HTTP header to make an authorized request to the Microsoft Graph:
header key | value |
---|---|
Authorization | Bearer <access-token> |
Add the following code to the ViewController
class:
See Microsoft Graph API to learn more about the Microsoft Graph API.
Use MSAL for Sign-out
Next, add support for sign-out.
Important
Signing out with MSAL removes all known information about a user from the application, as well as removing an active session on their device when allowed by device configuration. You can also optionally sign user out from the browser.
To add sign-out capability, add the following code inside the ViewController
class.
Enable token caching
By default, MSAL caches your app's tokens in the iOS or macOS keychain.
To enable token caching:
- Ensure your application is properly signed
- Go to your Xcode Project Settings > Capabilities tab > Enable Keychain Sharing
- Select + and enter one of the following Keychain Groups:
- iOS:
com.microsoft.adalcache
- macOS:
com.microsoft.identity.universalstorage
- iOS:
Add helper methods
Add the following helper methods to the ViewController
class to complete the sample.
iOS UI:
macOS UI:
For iOS only, get additional device information
Use following code to read current device configuration, including whether device is configured as shared:
Multi-account applications
This app is built for a single account scenario. MSAL also supports multi-account scenarios, but it requires some additional work from apps. You will need to create UI to help users select which account they want to use for each action that requires tokens. Alternatively, your app can implement a heuristic to select which account to use by querying all accounts from MSAL. For example, see accountsFromDeviceForParameters:completionBlock:
API
Test your app
Build and deploy the app to a test device or simulator. You should be able to sign in and get tokens for Azure AD or personal Microsoft accounts.
The first time a user signs into your app, they will be prompted by Microsoft identity to consent to the permissions requested. While most users are capable of consenting, some Azure AD tenants have disabled user consent, which requires admins to consent on behalf of all users. To support this scenario, register your app's scopes in the Azure portal.
After you sign in, the app will display the data returned from the Microsoft Graph /me
endpoint.
Next steps
Learn more about building mobile apps that call protected web APIs in our multi-part scenario series.
Question or issue on macOS:
I’m writing an iOS app that acts as, among other things, a telnet server. Naturally, it begins listening for connections as soon as it starts.
When I run the app in the Simulator, Mac OS X (I happen to be on 10.7.3) prompts me to Allow or Deny my application to accept incoming network connections. This is the standard Firewall message that Mac OS X uses for all unsigned, networked applications.
I grow weary of clicking “Allow” fifty or more times a day, and so I seek a way of permanently adding my app to the Firewall’s list of permitted apps.
I’ve tried the following.
In the last step there’s a significant decision. You could add either the .app application package, or Show Contents on that package and add the “Unix executable” within. I’ve tried both approaches.
Interestingly, Firewall will in fact stop warning you about the app—for a while. After a few runs, however—it isn’t clear to me what event actually causes this change, but it happens within half an hour or so for me, generally speaking—Firewall begins warning about the app again.
Ios Simulator Macbook
How do I set Firewall to permanently Allow my iOS app?
Naturally, I could bypass this whole problem by disabling the Mac OS X firewall. I could also avoid ever again getting a splinter in my foot by chopping it off. Neither of these courses of action recommend themselves to me.
What would you suggest?
How to solve this problem?
Solution no. 1:
So we want to suppress the following dialog
Do you want the application “NNN.app” to accept incoming network
connections?
which appear on every activation of the Xcode iOS simulator.
I believe there is now a solution for that. Basing my answer on this blog.
Mac Os Ios Image Download
Simply run the following commands in a Terminal window:
For me it worked. Please note simulator address is according to Xcode 8.
Solution no. 2:
After dabbling with this for some time, I found that manually adding the executable itself to the Firewall “Allow” list gives the desired result. You don’t add the .app, but rather the “Unix” executable inside the .app’s Contents folder. I believed I had tried this file before without success, but currently it’s working.
Solution no. 3:
I think the best solution might be to script the process of okaying your app to the firewall.
If I recall correctly, the latest OSX firewall is actually clever about identifying apps and fingerprints the allowed binaries. This prevents the surprisingly effective tactic of just naming your malware “system32.exe” &c to evade the firewall. If that’s the case, your app will be (correctly) blocked for not being the same binary that was okayed, and there’s not really any way around it.
So, try scripting the firewall allowing process and incorporate that into the build process.
Solution no. 4:
I never had luck with manually adding the executable to the firewall’s allowed-list. Here’s a solution using an automated mouse click:
- Download CLIclick. (Thank you Carsten.)
- Put it in a suitable location, say
/usr/local/bin
. - Get the
Allow
button’s screen coordinates using ⌘⇧4. (In my example, these are x: 750, y: 600.) Create a script with this content (the
w:
is the wait time in ms):(I couldn’t get
CLIclick
to work without “moving” it to the same location (them:+0,+0
part) and clicking again at the same spot withc:.
.)- Open Xcode’s
Preferences / Behaviors
and add the above script. - Enjoy!
Ios Simulator Macos Catalina
Solution no. 5:
Mac Os Ios Download
I don’t know if it is the right way but for me worked.
- Turn off the firewall
- Connect with the iphone app to your mac
- Check if everything in the connection working
- Turn on the firewall